Docker for Node.js Development: How to Create a Seamless Workflow
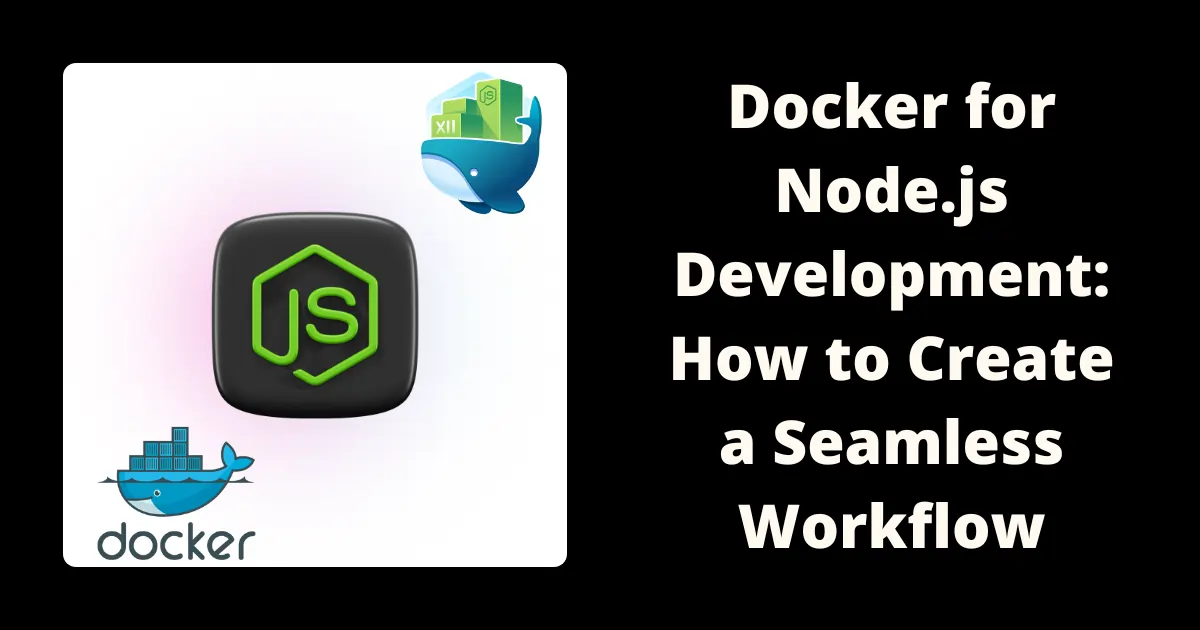
Overview
Table of Contents
- Introduction
- Why Use Docker for Node.js Development?
- Setting Up Docker for Node.js
- Use an official Node.js image as the base
- Set the working directory
- Copy package.json and package-lock.json
- Install dependencies
- Copy application code
- Expose the application port
- Command to run the app
- Docker Compose for Multi-Container Applications
- Best Practices for Using Docker with Node.js
- Build stage
- Production stage
Introduction
Docker has revolutionized how developers build, ship, and run applications. When combined with Node.js, Docker provides a reliable, isolated environment that ensures consistency across development, testing, and production stages. This blog will guide you on using Docker for Node.js development to create a seamless and efficient workflow.
Why Use Docker for Node.js Development?
Docker simplifies the development process by packaging your application and its dependencies into lightweight, portable containers. Here’s why it’s beneficial:
- Consistency: Eliminates "works on my machine" issues by standardizing environments.
- Scalability: Simplifies scaling applications across multiple containers.
- Efficiency: Speeds up development with isolated environments and easy dependency management.
- Portability: Containers can run anywhere—on your laptop, a server, or the cloud.
Setting Up Docker for Node.js
1. Install Docker
First, install Docker on your machine. You can download it from Docker’s official website. Follow the installation instructions for your operating system.
2. Create a Node.js Application
Set up a simple Node.js application.
Example of a basic app.js
file:
const express = require("express");
const app = express();
const port = 3000;
app.get("/", (req, res) => {
res.send("Hello, Docker with Node.js!");
});
app.listen(port, () => {
console.log(`App running at http://localhost:${port}`);
});
Create a package.json file with your application dependencies:
npm init -y
npm install express
3. Write a Dockerfile
A Dockerfile contains the instructions to build a Docker image for your application.
Example Dockerfile:
# Use an official Node.js image as the base
FROM node:18-alpine
# Set the working directory
WORKDIR /usr/src/app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy application code
COPY . .
# Expose the application port
EXPOSE 3000
# Command to run the app
CMD ["node", "app.js"]
4. Create a .dockerignore File
A .dockerignore
file specifies files and directories to exclude when building your Docker image.
Example .dockerignore
file:
node_modules
npm-debug.log
5. Build and Run the Docker Container
1. Build the Docker image:
docker build -t nodejs-docker-app .
2.Run the Docker container:
docker run -p 3000:3000 nodejs-docker-app
3. Visit http://localhost:3000 in your browser.
You should see the message: Hello, Docker with Node.js!
Docker Compose for Multi-Container Applications
For more complex applications, you might need a database, cache, or other services. Use Docker Compose to manage multiple containers.
Example docker-compose.yml
File
Here’s an example with Node.js and MongoDB:
version: '3.8'
services:
app:
build: .
ports:
- "3000:3000"
volumes:
- .:/usr/src/app
depends_on:
- db
db:
image: mongo
container_name: mongodb
ports:
- "27017:27017"
1. Start the application:
docker-compose up
2. Both Node.js and MongoDB will run in isolated containers, accessible on the specified ports.
Best Practices for Using Docker with Node.js
1. Use Multi-Stage Builds
Reduce image size by separating build and runtime stages.
# Build stage
FROM node:18-alpine as builder
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
# Production stage
FROM node:18-alpine
WORKDIR /usr/src/app
COPY --from=builder /usr/src/app/dist ./dist
EXPOSE 3000
CMD ["node", "dist/app.js"]
2. Optimize Docker Images
Use lightweight base images like node:18-alpine to minimize image size.
3. Use Volumes During Development
Map your local directory to the container for real-time code changes:
volumes:
- .:/usr/src/app
4. Monitor Container Performance
Use tools like Docker Stats or third-party monitoring solutions to track container resource usage.
Frequently Asked Questions
1. Why should I use Docker for Node.js development?
Docker ensures consistent environments, simplifies dependency management, and makes your application portable and scalable.
2. Can I use Docker with other Node.js tools?
Yes, you can integrate Docker with tools like PM2, Redis, or databases to enhance your Node.js workflows.
3. How do I scale a Node.js app with Docker?
Use Docker Compose for multiple containers or Docker Swarm/Kubernetes for container orchestration.
4. Does Docker slow down development?
No, Docker accelerates development by providing isolated, predictable environments and real-time synchronization during local development.
5. Is Docker suitable for small projects?
Yes, even small projects benefit from Docker’s consistency and ease of deployment, though the overhead might be unnecessary for very simple applications.